Django 기본 CBV API (Generic date views)
2022. 1. 11. 15:01ㆍ강의 정리/Django Views
반응형
장고(Django)를 배우기 시작한 입문자이시거나, 또는 배우고 싶은 생각이 있으신 분은 위 출처의 강의를 적극 추천드립니다!!!
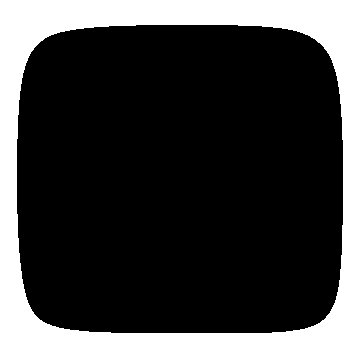
- Generic Date View
- ArchiveIndexView: 지정 날짜필드 역순으로 정렬된 목록
- YearArchiveView: 지정 year 년도의 목록
- MonthArchiveView: 지정 year/month 월의 목록
- WeekArchiveView: 지정 year/week 주의 목록
- DayArchiveView: 지정 year/month/day 일의 목록
- TodayArchiveView: 오늘 날짜의 목록
- DateDetailView: 지정 year / month / day 목록 중에서 특정 pk의 detail
- DetailView와 비교하여, URL에 날짜(year / month / day)를 쓰고자 할 경우에 유용하다.
# 공통 옵션
allow_future (디폴트 : False) : 미래의 시간을 허용하겠느냐
- False : 현재 시간 이후의 Record는 제외
ArchiveIndexView: 지정 날짜필드 역순으로 정렬된 목록
- 최신순으로 목록을 보고자 할 때
- 필요한 URL 인자 : 없음
- 옵션
- model
- date_field : 정렬 기준 필드
- date_list_period (디폴트 : "year")
- 디폴트 template_name_suffix : "모델명_archive.html"
- Context
- latest : QuerySet
- date_list : 등록된 Record의 년도 목록 ex) Year단위로 글들을 그룹핑해서 list로 제공해줌
# instagram/views.py
from django.views.generic import ArchiveIndexView
post_archive = ArchiveIndexView.as_view(model=Post, date_field='created_at')
# instagram/urls.py
urlpatterns = [
path('post_archive/', views.post_archive, name='post_archive'),
]
# instagram/templates/instagram/post_archive.html
- Context : 템플릿에서 사용할 수 있는 객체
- latest : QuerySet
- date_list : 등록된 Record의 년도 목록 ex) Year단위로 글들을 그룹핑해서 list로 제공해줌
<h2>latest</h2>
{{ latest }}
<h2>date_list</h2>
{{ date_list }}
# 데이터들의 연도와 월을 랜덤하게 지정해주는 방법
python manage.py shell
>>> from instagram.models import Post
>>> post_list = Post.objects.all()
>>> import random
>>> for post in post_list:
... year = random.choice(range(1990, 2020))
... month = random.choice(range(1, 13))
... post.created_at = post.created_at.replace(year=year, month=month)
... post.save()
>>> 랜덤하게 바꾼 결과
>>> Post.objects.all().values_list('created_at__year')
<QuerySet [(1990,), (2009,), (1998,), (1993,), (2012,), (1991,), (1991,), (2010,), (1997,), (2005,), (2009,), (2015,), (1998,), (2003,), (2000,), (1996,), (1990,), (2004,), (2019,), (1991,), '...(remaining elements truncated)...']>
튜플을 리스트로 평평하게 변환
>>> Post.objects.all().values_list('created_at__year', flat=True)
<QuerySet [1990, 2009, 1998, 1993, 2012, 1991, 1991, 2010, 1997, 2005, 2009, 2015, 1998, 2003, 2000, 1996, 1990, 2004, 2019, 1991, '...(remaining elements truncated)...']>
직전 결과를 변수에 담아오기
year_list = _
>>> set(year_list)
{1990, 1991, 1992, 1993, 1994, 1995, 1996, 1997, 1998, 1999, 2000, 2001, 2002, 2003, 2004, 2005, 2006, 2007, 2008, 2009, 2010, 2011, 2012, 2013, 2014, 2015, 2016, 2018, 2019}
YearArchiveView: 지정 year 년도의 목록
- 필요한 URL 인자 : "year"
- 옵션
- model, date_field
- date_object_list (디폴트 : False) : 거짓일 경우, object_list를 비움
- date_list_period (디폴트 : "month") : 지정 년도에서 month 단위로 Record가 있는 날짜 리스트
- 디폴트 template_name_suffix : "모델명_archive_year.html"
- Context
- year, previous_year, next_year
- date_list : 등록된 Record의 월도 목록 ex) Month단위로 글들을 그룹핑해서 list로 제공해줌
- object_list : 같은 년도를 가진 객체들
# instagram/views.py
from django.views.generic.dates import YearArchiveView
post_archive_year = YearArchiveView.as_view(model= Post, date_field='created_at', make_object_list=True)
# instagram/urls.py
urlpatterns = [
path('archive/<year:year>/', views.post_archive_year, name='post_archive_year'),
]
# instagram/templates/instagram/post_archive_year.html
- Context : 템플릿에서 사용할 수 있는 객체
- latest : QuerySet
- date_list : 등록된 Record의 년도 목록 ex) Year단위로 글들을 그룹핑해서 list로 제공해줌
<h2> year </h2>
{{ year }}
<h2> previous year </h2>
{{ previous_year }}
<h2> next year </h2>
{{ next_year }}
<h2> object list </h2>
{{ object_list }}
MonthArchiveView: 지정 year/month 월의 목록
- 필요한 URL 인자 : "year", "month"
- 옵션
- model, date_field
- date_field (디폴트 : "%b") :
- 숫자 포맷은 "%m"
- 디폴트 template_name_suffix : "모델명_archive_month.html"
- Context : 템플릿에서 사용할 수 있는 객체
- month, previous_month, next_month
- date_list : 등록된 Record의 날짜 목록 ex) 날짜 단위로 글들을 그룹핑해서 list로 제공해줌
- object_list : 같은 월을 가진 객체들
# instagram/urls.py
urlpatterns = [
path('archive/<year:year>/<month:month>/', views.post_archive_month, name='post_archive_month'),
]
# instagram/templates/instagram/post_archive_month.html
DayArchiveView: 지정 year/month/Day 일의 목록
- 필요한 URL 인자 : "year", "month", "day"
- 옵션
- model, date_field
- month_format (디폴트 : "%b") :
- 숫자 포맷은 "%m"
- 디폴트 template_name_suffix : "모델명_archive_day.html"
- Context : 템플릿에서 사용할 수 있는 객체
- day, previous_day, next_day
- date_list : 등록된 Record의 날짜 목록 ex) 날짜 단위로 글들을 그룹핑해서 list로 제공해줌
- object_list : 같은 날짜를 가진 객체들
TodayArchiveView
- 필요한 URL 인자 : 없음
- DayArchiveView와 유사하게 동작하지만,
- year/month/day 인자를 받지 않음
- previous_day, next_day 미제공
DateDetailView : 지정 year/month/day 목록 중에서 특정 pk의 detail
- 필요한 URL 인자 : "year", "month", "day", "pk"(or "slug")
반응형
'강의 정리 > Django Views' 카테고리의 다른 글
URL Reverse를 통해서 유연하게 URL 생성하기 (0) | 2022.01.11 |
---|---|
적절한 HTTP 상태코드로 응답하기 (0) | 2022.01.11 |
Django 뷰 장식자(Decorators) (0) | 2022.01.10 |
Django 기본 CBV API (Generic display views) (0) | 2022.01.10 |
Django 기본 CBV API (Base views) (0) | 2022.01.09 |