2022. 2. 15. 15:38ㆍ카테고리 없음
장고(Django)를 배우기 시작한 입문자이시거나, 또는 배우고 싶은 생각이 있으신 분은 위 출처의 강의를 적극 추천드립니다!!!
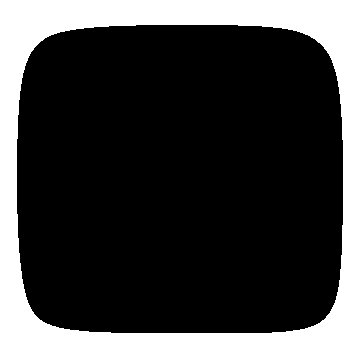
Django 유저 페이지 구현하기
#insta/urls.py
from django.urls import path, re_path
from django.contrib.auth.validators import UnicodeUsernameValidator
from . import views
# reverse에 사용할 이름
app_name = 'insta'
# 주소에 오는 username이 Unicode 형태인지 확인하는 Validator
# username_regax = UnicodeUsernameValidator.regex.lstrip('^').strip('$')
urlpatterns = [
path('post/new', views.post_new, name='post_new'),
path('post/<int:pk>', views.post_detail, name='post_detail'),
re_path(r'^(?P<username>[\w.@+-]+)/$', views.user_page, name='user_page'),
]
@deconstructible
class UnicodeUsernameValidator(validators.RegexValidator):
regex = r"^[\w.@+-]+\Z"
message = _(
"Enter a valid username. This value may contain only letters, "
"numbers, and @/./+/-/_ characters."
)
flags = 0
https://github.com/django/django/blob/main/django/contrib/auth/validators.py
#UnicodeUsernameValidator은 username이 Unicode 형태의 문자인지 검사하는 Validator입니다.
https://docs.djangoproject.com/en/4.0/ref/contrib/auth/
django.contrib.auth | Django documentation | Django
Django The web framework for perfectionists with deadlines. Overview Download Documentation News Community Code Issues About ♥ Donate
docs.djangoproject.com
#정규 표현식에 익숙해지자!
07-2 정규 표현식 시작하기
[TOC] ## 정규 표현식의 기초, 메타 문자 정규 표현식에서 사용하는 메타 문자(meta characters)에는 다음과 같은 것이 있다. > ※ 메타 문자란 원래 ...
wikidocs.net
#accounts/forms.py
# username 중복 방지 함수
def clean_username(self):
username = self.cleaned_data.get('username')
if username:
qs = User.objects.filter(username='post')
if qs.exists():
raise forms.ValidationError("지정할 수 없는 아이디입니다.")
return username
# username이 혹시 다른 url의 이름과 겹치면 오류가 발생하기에 사전에 방지해줍니다.
#insta/views.py
# 유저 페이지
def user_page(request, username):
page_user = get_object_or_404(get_user_model(), username=username, is_active=True)
# 해당 유저가 쓴 글만을 filter 처리하여서 저장
post_list = Post.objects.filter(author=page_user)
return render(request, "insta/user_page.html", {
"page_user": page_user,
"post_list": post_list,
})
#get_object_or_404의 코드입니다.
def get_object_or_404(klass, *args, **kwargs):
"""
Use get() to return an object, or raise an Http404 exception if the object
does not exist.
klass may be a Model, Manager, or QuerySet object. All other passed
arguments and keyword arguments are used in the get() query.
Like with QuerySet.get(), MultipleObjectsReturned is raised if more than
one object is found.
"""
queryset = _get_queryset(klass)
if not hasattr(queryset, "get"):
klass__name = (
klass.__name__ if isinstance(klass, type) else klass.__class__.__name__
)
raise ValueError(
"First argument to get_object_or_404() must be a Model, Manager, "
"or QuerySet, not '%s'." % klass__name
)
try:
return queryset.get(*args, **kwargs)
except queryset.model.DoesNotExist:
raise Http404(
"No %s matches the given query." % queryset.model._meta.object_name
)
#is_active는 접근이 허용된 사람만 확인하게 해주는 옵션입니다.
#insta/templates/insta/user_page.html
{% extends "insta/layout.html" %}
{% block content %}
<div class="container">
<div class="row pt-5 pb-5">
<div class="col-sm-3" style="text-align: center;">
<img src="{{ page_user.profile_url }}" class="rounded-circle" style="width: 160px;" />
</div>
<div class="col-sm-9">
{{ page_user.username }}
<a href="{% url "profile_edit" %}" class="btn btn-secondary btn-sm">
Edit Profile
</a>
<hr/>
0 posts, 0 followers, 0 following
<hr/>
{{ page_user.name }}
</div>
</div>
<div class="row mt-3">
{% for post in post_list %}
<div class="col-sm-4">
<img src="{{ post.photo.url }}" alt="{{ post.caption }}" style="width: 100%;%" />
</div>
{% endfor %}
</div>
</div>
{% endblock %}