Django 포스팅 detail view 구현
2022. 2. 14. 17:21ㆍ카테고리 없음
반응형
장고(Django)를 배우기 시작한 입문자이시거나, 또는 배우고 싶은 생각이 있으신 분은 위 출처의 강의를 적극 추천드립니다!!!
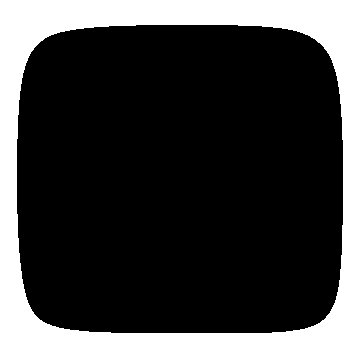
Django 포스팅 detail view 구현하기
#insta/urls.py
from django.urls import path
from . import views
app_name = 'insta'
urlpatterns = [
path('post/new', views.post_new, name='post_new'),
path('post/<int:pk>', views.post_detail, name='post_detail'),
]
#insta/views.py
from django.shortcuts import redirect, get_object_or_404
# 포스팅 detail
def post_detail(request, pk):
# 해당 pk의 포스팅 객체가 없을 경우 404 에러 반환
post = get_object_or_404(Post, pk=pk)
return render(request, "insta/post_detail.html", {
"post" : post,
})
# 항상 디테일을 구현하면 관련 모델에 get_absolute_url을 지정해주어야한다.
#insta/models.py
class Post(models.Model):
author = models.ForeignKey(settings.AUTH_USER_MODEL, on_delete=models.CASCADE)
photo = models.ImageField(upload_to="insta/post/%Y%m%d")
# 내용
caption = models.TextField()
tag_set = models.ManyToManyField('Tag', blank=True)
location = models.CharField(max_length=100)
# detail view
def get_absolute_url(self):
return reverse("insta:post_detail", args=[self.pk])
# 만약 이름으로 접근하게 되면 이름 형태로 반환해주게끔 accounts/models.py에서 설정해준다.
@property
def name(self):
return f"{self.first_name} {self.last_name}"
# 유저가 프로필이 있으면, 유저의 프로필을 보여주고, 없으면 기본 프로필을 보여주는 함수를 accounts/models.py에서 설정해준다.
# profile이 있는지 검사하고 알맞은 값을 반환하는 로직
@property
def profile_url(self):
if self.profile:
return self.profile.url
else:
return resolve_url('pydenticon_image', self.username)
#insta/templates/insta/post_detail.html
{% extends "insta/layout.html" %}
{% load bootstrap4 %}
{% block content %}
<div class="container">
<div class="row">
<div class="col-sm-12">
<div class="card">
<div class="card-header">
<img src= "{{ user.profile_url }}" style="width: 24px; height: 24px;"/>
{{ post.author }}
</div>
<div class="card-body">
{% if post.photo.url %}
<img src="{{ post.photo.url }}" style="width: 100%;"/>
{% endif %}
</div>
<div class="card-footer">
{% for tag in post.tag_set.all %}
<span class="badge badge-primary" style="color: #fff;
background-color: #007bff;">
#{{ tag.name }}
</span>
{% endfor %}
</div>
</div>
</div>
</div>
</div>
{% endblock %}
반응형